In this course we are going to make a game, we chose the concept “Suit’em up” which is a game where you are going to, as Barney the robot, cleanse a suit from bugs which has infested it.
The first task I got from my lead programmer was to fix the collision system for the walls. We have discussed different options; we discussed alpha collision, some sort of tile collision, square collision and line collision. With the alpha collision we were going to add a layer with black and white colors where you can walk on the white but not on the black, we dismissed this idea pretty quickly as our teacher told us it would not be optimal. The tile collision we also dismissed quite quickly because there would have to be so many different tiles with different looks and they would have to be very small. We should probably not be able to use the same tile that many times, it would not be the most optimal solution either. With the square collision there would be too many small squares and really not the easiest to program since the terrain is not that straight. The walls of the different rooms will look something like in this photo:
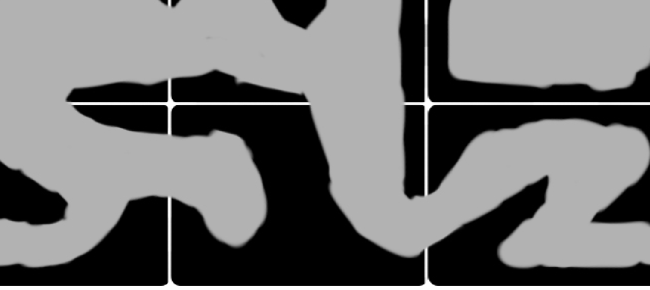
So we finally discussed the line collision. We talked about making a tool where we can set out different points along the walls and check the collision between them. So I made a prototype of this tool and it worked fine. So we thought we would give the line collision a try.
The prototype I made of the point-and-click-to-make-a-txt-file-with-points is written in c# and I used Windows Forms and it look something like this:
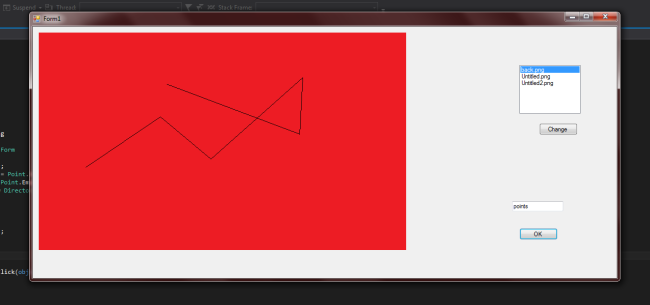
You can choose the background (preferably the picture of the room you want to put the points in), then click to set the points and save them in a txt-file with the name of your choice (preferably the name of the room). Then you just read the file in your game-code and save them in a vector to iterate through them to check the collision.
When I first started looking at the math it looked impossible. But I ❤ Google and after some searching I found this site.
Basic Collision Detection in 2D – Part 2
Which gave me almost exactly what I needed.

This is collision between a circle (our player and enemies will have colliders which are circles) and an infinite line. All I had to add was the code that checked if the collision point was inside the outer points of the lines. As it is now I think it is not optimized but if we set in stone that we will use the line collision I will look in to it some more.
So I made a prototype of the collision system as well. With code from the site I mentioned earlier.
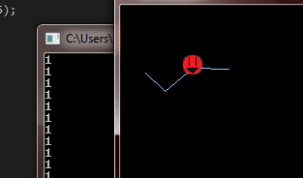
This is in SDL though, so I have to rewrite it with SFML and then implement it to the game.